Defining and Calling a Function in Python
What is a function?
It’s a block of statements that performs a specific task, which can be later reused any number of times in the program. It also helps to reduce the number of lines in the code and make it looks readable
Python already has a whole bunch of built-in functions that we have been using
For example:
print()
Print function get the input (whatever is specified inside the parenthesis) either a string or variable and print that to the console
len()
len function, get the input specified in the parenthesis either string or list, and helps to find the length
int()
int function helps to convert the input specified in the parenthesis and convert the values to an integer
So, Let’s talk about, how to define a function with a simple example:
To define a function, use the below syntax with proper indentation
def my_function(): print("created my 1st function")
Now, We have created a function, This code will not be executed unless the function is called in any part of the code.
To call a function
my_function()
This will call the above-defined function and execute the code inside the block
Output:
created my 1st function
How functions are useful to reduce typing code
I have been using the example in the “reeborg.ca“, Where we need to write a code So that the robot can go to the goal location: just like a map
In this example, We need to create a new function or call a pre-defined function to move the robot to escape all the hurdles and reach the Goal
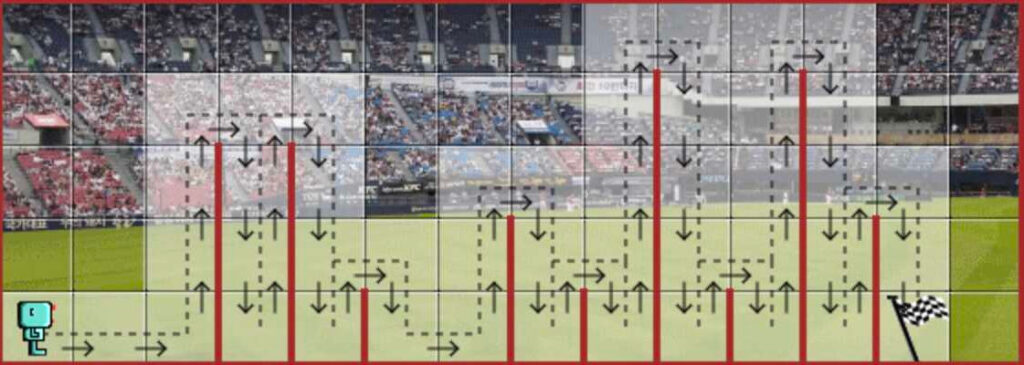
Below is the actual piece of code, That helps the Robot to move and jump the huddles
def turn_right(): turn_left() turn_left() turn_left() def huddle(): turn_left() while wall_on_right(): move() turn_right() move() turn_right() while not wall_in_front(): move() turn_left() while not at_goal(): if not front_is_clear(): huddle() else: move()
Written a code help to escape from the huddle and reaches the goal below
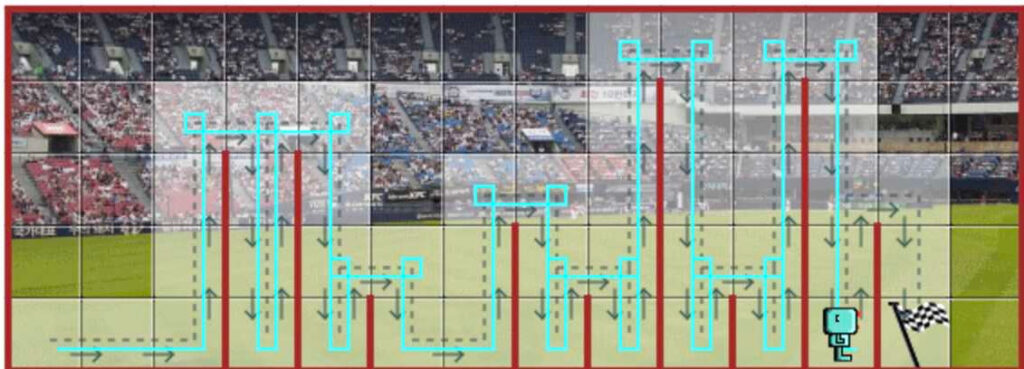
Let’s think about the same scenarios without function, Yes, We need to manually write all the steps which can go beyond 100 steps,
Now the program is completed within 17 steps and is more readable
Also, You may notice the program looks indented, Yes, Indentation is very much needed in python and if it is not defined properly you may end up messing up the program flow
For example:
def myfunction() print(“1st ”) print(“2nd”) myfunction()
When you call the function, you will get an output below
Output:
1st 2nd
So, If you define your function below
def myfunction() print(“1st ”) print(“2nd”) myfunction()
Your output will be as below
1st
Yes, In Python if there is no space or tab below each “function”, “loop” or “if condition” it is considered not to be part of the function or loop, So be careful on defining the functions
Let’s see, how to pass an argument inside a function:
def my_function(arg1, arg2): #Define your function here
example:
def addition(a,b) print(a+b) addition (10,20)
Output :
30
Conclusion:
Congratulations, you have learned about functions in Python and also why indentation is essential in a program. Hope you guys had a good time reading this. Check here for more Python learning series blogs. Good Luck!