Python program to iterate through two lists in parallel
Have you ever needed to work with two lists of data in Python, and wanted to process them at the same time? Fortunately, Python provides several ways to iterate through two lists in parallel, allowing you to work with both sets of data simultaneously.
Iterating through two lists in parallel is a common task in Python, and can be accomplished using various methods such as the zip and enumerate functions, as well as a while loop with indexing. These approaches allow you to process elements from both lists simultaneously, with some methods able to handle lists of different sizes.
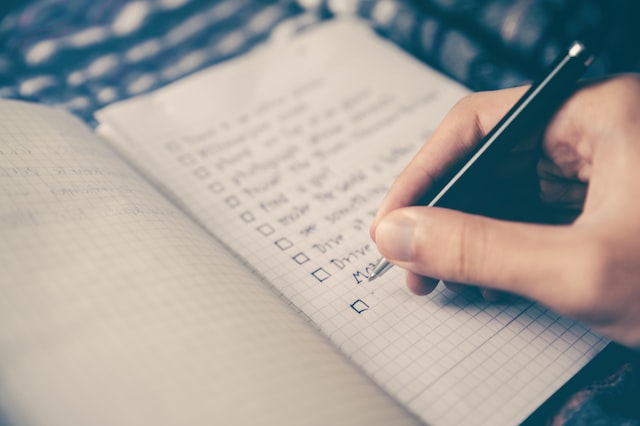
Introduction
Python is a high-level programming language widely used for scripting, web development, data analysis, and artificial intelligence. It is known for its simplicity and ease of use, making it a popular choice among beginners and seasoned developers alike. One of the most common operations in programming is iterating through lists, and sometimes it is necessary to iterate through two lists in parallel. In this article, we will discuss how to achieve this using Python.
Iterating through Two Lists in Parallel (Equal & Different Lengths)
We will see the multiple ways to iterate two lists both equal length and different lengths lists
Using Zip Function
Iterating through two Equal Size lists
Iterating through two lists in parallel means traversing two lists at the same time, comparing or performing operations on corresponding elements. In Python, we can achieve this by using the built-in zip function. The zip function takes two or more iterable objects and returns a new iterator that aggregates elements from each of the iterables.
Syntax:
zip(*iterables)
Where *iterables is a variable length argument that can accept two or more iterable objects.
Example
Let us consider two lists of equal length:
list1 = [1, 2, 3, 4] list2 = ['a', 'b', 'c', 'd'] for item1, item2 in zip(list1, list2): print(item1, item2)
Output:
(1, 'a') (2, 'b') (3, 'c') (4, 'd')
In the above example, the zip function creates an iterator that aggregates elements from both list1 and list2. The for loop then iterates through each pair of corresponding elements in the iterator and assigns them to the variables item1 and item2. We can then perform operations on these items as required.
Iterating through two different Size lists
It is important to note that the zip function stops iterating as soon as one of the iterables is exhausted. This means that if the two lists being zipped have different lengths, the shorter list will determine the number of iterations.
Example:
list1 = [1, 2, 3] list2 = ['a', 'b', 'c', 'd'] for item1, item2 in zip(list1, list2): print(item1, item2)
Output:
(1, 'a') (2, 'b') (3, 'c')
Try it yourself -> Online Python editor to test the example
In the above example, the zip function stops iterating after the third iteration since list1 has only three elements. The fourth element in list2 is not included in the iteration.
Using enumerate Function
Iterating through two Equal Size lists
Iterate through two lists in parallel in Python. One alternative method is to use the built-in enumerate function along with a for loop to iterate over the indices of the two lists.
Example:
# create two lists of the same size list1 = [1, 2, 3, 4] list2 = ['a', 'b', 'c', 'd'] # iterate through the lists in parallel using enumerate for i, (item1, item2) in enumerate(zip(list1, list2)): print(f"Index {i}: {item1} {item2}")
Output:
Index 0: 1 a Index 1: 2 b Index 2: 3 c Index 3: 4 d
Try it yourself -> Online Python editor to test the example
In this example, we first created two lists, list1, and list2, of the same size. We then used the zip function to create an iterator that aggregates elements from each of the two input lists, and the enumerate function to generate a sequence of index-value pairs over which we can iterate. The for loop iterates over the index-value pairs, unpacking each pair into the variables i, item1, and item2, respectively, and prints them to the console.
Using enumerate along with zip allows us to iterate through two lists in parallel and also access their indices at the same time. This can be useful in situations where we need to reference the indices of the input lists.
However, it’s important to note that this method may be slightly less efficient than using zip alone, as it involves an additional step of generating the index-value pairs using enumerate.
Iterating through two different Size lists
use enumerate to iterate through two lists of different sizes. When using zip to iterate over multiple lists in parallel, the iteration stops as soon as the shortest list is exhausted. However, when using enumerate, the loop will continue until the end of the long list is reached, and any missing elements in the shorter list will be skipped.
Example:
# create two lists of different sizes list1 = [1, 2, 3] list2 = ['a', 'b', 'c', 'd'] # iterate through the lists in parallel using enumerate for i, (item1, item2) in enumerate(zip(list1, list2)): print(f"Index {i}: {item1} {item2}")
Output:
Index 0: 1 a Index 1: 2 b Index 2: 3 c Try it yourself -> Online Python editor to test the example
It is the same as the above example for two lists of the same length, But you might have noticed that the loop stops after the third iteration because the list1 has only three elements, while list2 has four elements. The fourth element in list 2 (‘d’) is not printed because it has no corresponding element in list 1.
Using While Loop
Iterate through two lists in parallel in Python is to use a while loop along with indexing
Example:
# create two lists of the same size list1 = [1, 2, 3, 4] list2 = ['a', 'b', 'c', 'd'] # iterate through the lists in parallel using a i = 0 while i < len(list1) and i < len(list2): print(list1[i], list2[i]) i += 1
Output:
(1, 'a') (2, 'b') (3, 'c') (4, 'd')
Try it yourself -> Online Python editor to test the example
In this example, we first created two lists, list1, and list2, of the same size. We then initialized a counter variable i to zero and used a while loop to iterate over the indices of the two lists. The while loop continues as long as the counter variable i is less than the length of both input lists. Inside the loop, we print the elements at the corresponding indices in list1 and list2, respectively, and increment the counter variable i.
Using a while loop along with indexing allows us to iterate through two lists in parallel without using any built-in Python functions, but it may be slightly less concise and less efficient than using zip or enumerate in most cases.
Conclusion
Iterating through two lists in parallel is a common operation in programming, and Python provides an easy way to achieve this using the zip, enumerate function, or using loop. By using these methods, we can compare or perform operations on corresponding elements from two or more iterable objects. It is important to select the above methods based on the use case and performance.
Good Luck with your Learning !!